This project is a demo of how to use AG Grid with a Next.js application. AG Grid is a powerful and flexible JavaScript data grid that provides advanced features like sorting, filtering, and pagination.
This project demonstrates how to fetch data from a remote API and display it in an AG Grid table. The table allows users to filter and paginate the data, making it easy to work with large data sets.
Project Demo
https://ag-grid-demo-sand.vercel.app/
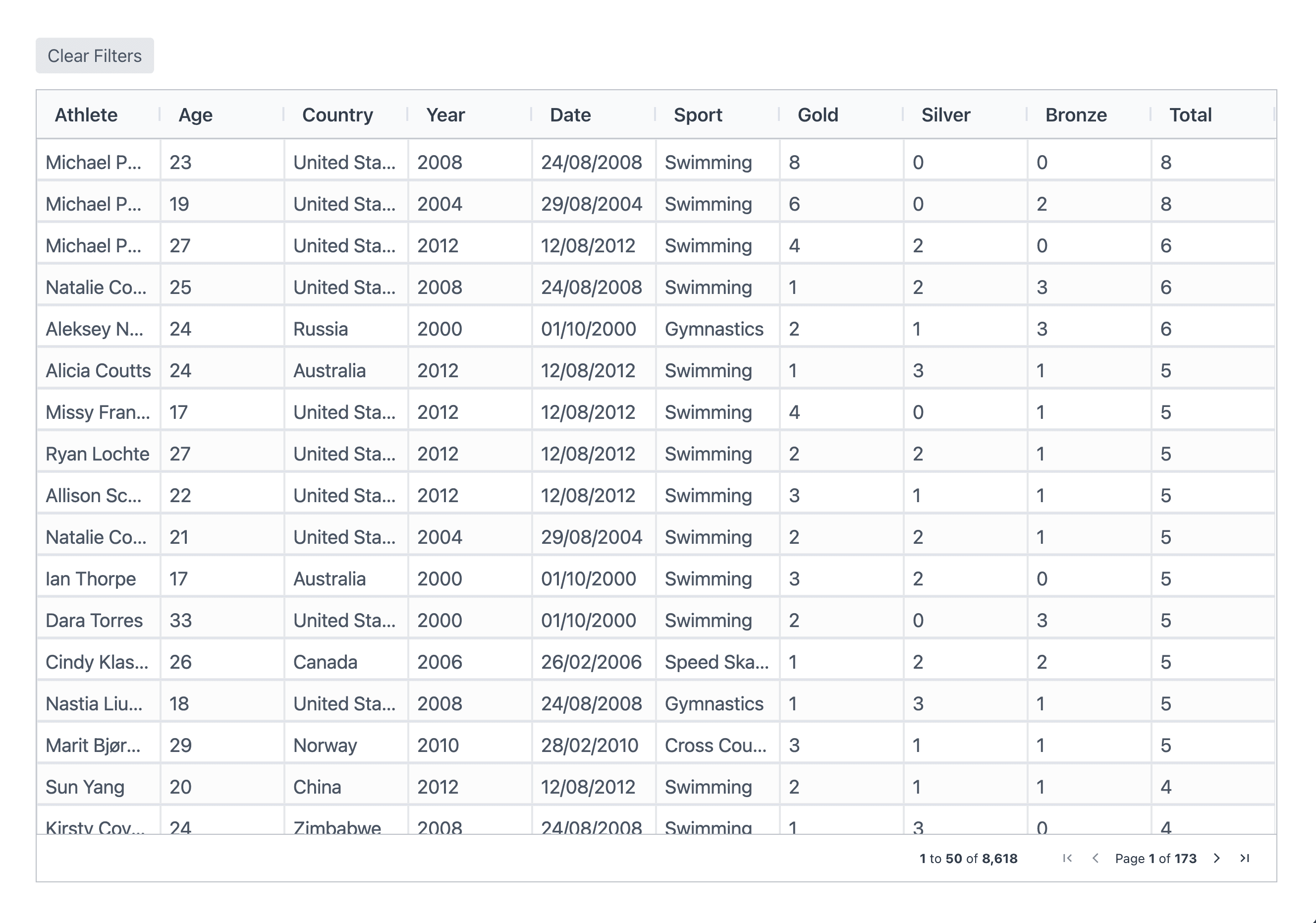
Github repository
https://github.com/owolfdev/ag-grid-demo
Getting Started
To run this project, you will need Node.js installed on your machine. Once you have Node.js installed, follow these steps:
- Clone the repository to your local machine.
- Navigate to the project directory in your terminal.
- Run npm install to install the project dependencies.
- Run npm run dev to start the development server.
- Open http://localhost:3000 in your browser to view the demo.
AG Grid Component
The AG Grid component in this demo is a functional component written in TypeScript. It fetches data from a remote API using the useEffect hook and sets it as the rowData state variable.
The columnDefs variable defines the columns to display in the table. It specifies the field name and the filter type for each column.
const columnDefs: agGrid.ColDef[] = [
{ field: "athlete", filter: "agTextColumnFilter" },
{ field: "age", filter: "agNumberColumnFilter" },
{ field: "country", filter: "agSetColumnFilter" },
{ field: "year", filter: "agNumberColumnFilter" },
{ field: "date", filter: "agDateColumnFilter" },
{ field: "sport", filter: "agTextColumnFilter" },
{ field: "gold", filter: "agNumberColumnFilter" },
{ field: "silver", filter: "agNumberColumnFilter" },
{ field: "bronze", filter: "agNumberColumnFilter" },
{ field: "total", filter: "agNumberColumnFilter" },
];
The defaultColDef variable sets default properties for all columns. In this demo, it sets the flex, minWidth, and resizable properties.
const defaultColDef: agGrid.ColDef = {
flex: 1,
minWidth: 100,
resizable: true,
};
The onGridReady function sets the gridApi and gridColumnApi state variables when the grid is ready. This allows us to interact with the grid through the API.
const onGridReady = (params: agGrid.GridReadyEvent) => {
setGridApi(params.api);
setGridColumnApi(params.columnApi);
};
The onPaginationChanged function is called when the pagination changes. In this demo, it simply logs a message to the console.
const onPaginationChanged = () => {
console.log("onPaginationChanged");
};
The clearFilters function clears all filters when the "Clear Filters" button is clicked.
const clearFilters = () => {
if (gridApi) {
gridApi.setFilterModel(null);
}
};
The component returns an AgGridReact component with the necessary properties to display the grid. It also includes some styles to customize the appearance of the grid and the pagination controls.
return (
<div className="ag-theme-alpine grid-container">
<div className="flex justify-between align-middle mb-4 text-lg text-[#4b5563] ">
<button
className="rounded bg-gray-200 px-3 py-1"
onClick={clearFilters}
>
Clear Filters
</button>
</div>
<AgGridReact
className="ag-grid"
columnDefs={columnDefs}
rowData={rowData}
defaultColDef={defaultColDef}
onGridReady={onGridReady}
pagination={true}
paginationPageSize={50}
onPaginationChanged={onPaginationChanged}
></AgGridReact>
<style jsx global>{`
.grid-container {
height: 800px;
width: 100%;
}
.ag-grid .ag-cell {
padding: 0.5rem;
font-size: 1.2rem;
color: #4b5563;
border-color: #e5e7eb;
line-height: 1.5;
}
.ag-grid .ag-header-cell {
font-weight: 600;
font-size: 1.2rem;
color: #374151;
background-color: #f9fafb;
border-color: #e5e7eb;
}
`}</style>
</div>
);
};
Conclusion
This demo shows how easy it is to use AG Grid with a Next.js application. AG Grid provides powerful data grid features that make it easy to work with large data sets.